[Spring] Welcome Page 만들기
- spring initializer 이용하여 spring 프로젝트 생성한다.
아래의 링크를 클릭하여 이동하고, 이미지와 동일하게 설정한 후 Generate 를 클릭해준다.
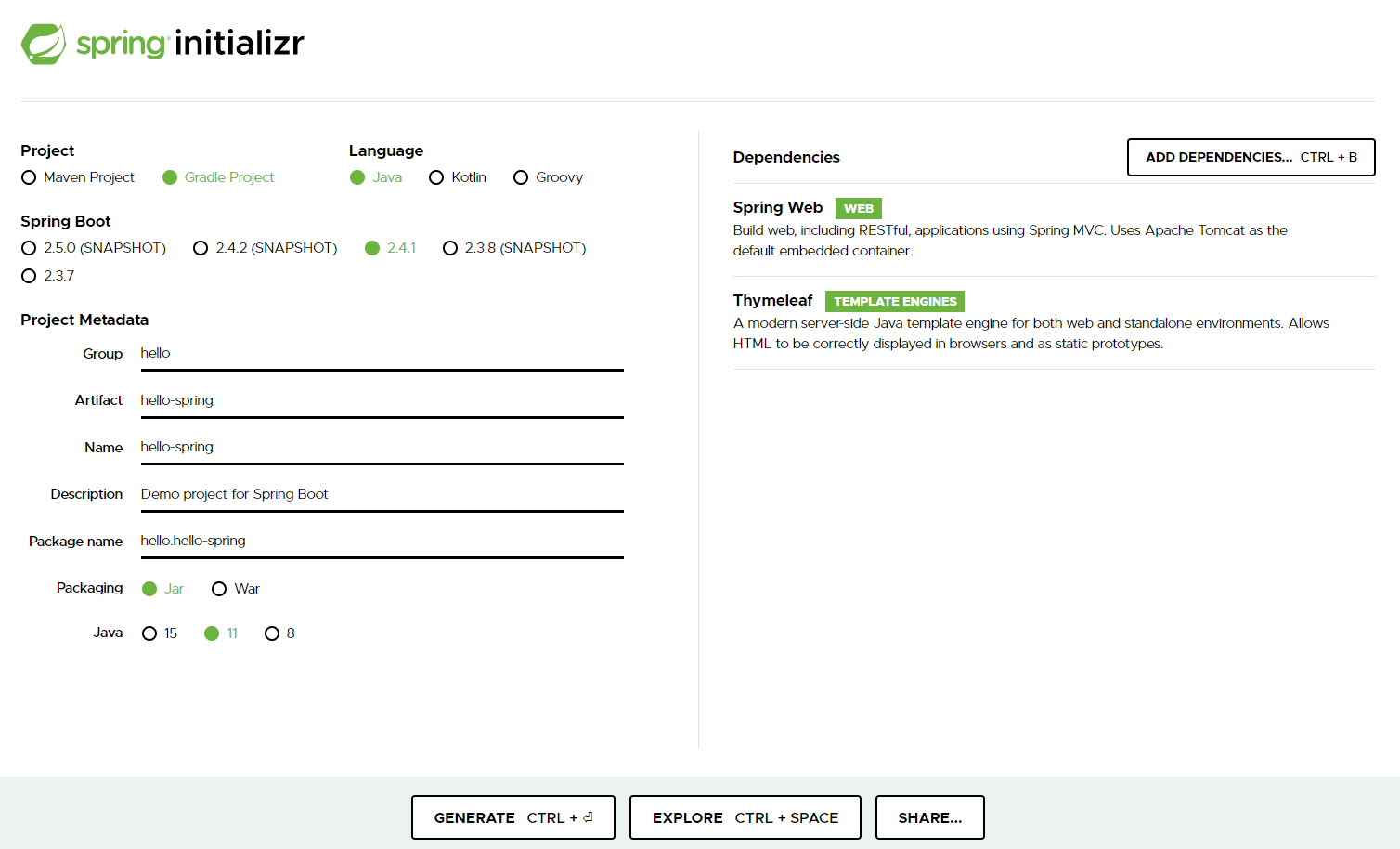
- 다운을 받은 후, 압축을 해제해준다.
- IntelliJ에서 build.gradle 을 Project로 열어준다.
src > main > java > hello.hellospring > HelloSpringApplication
해당 위치에 아래와 같은 파일이 생성되었음을 확인할 수 있다.
1
2
3
4
5
6
7
8
9
10
11
12
13
|
package hello.hellospring;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class HelloSpringApplication {
public static void main(String[] args) {
SpringApplication.run(HelloSpringApplication.class, args);
}
}
|
cs |
- Run을 해준다.
아래 화면과 같이 나오면서 실행이 될 것이다.
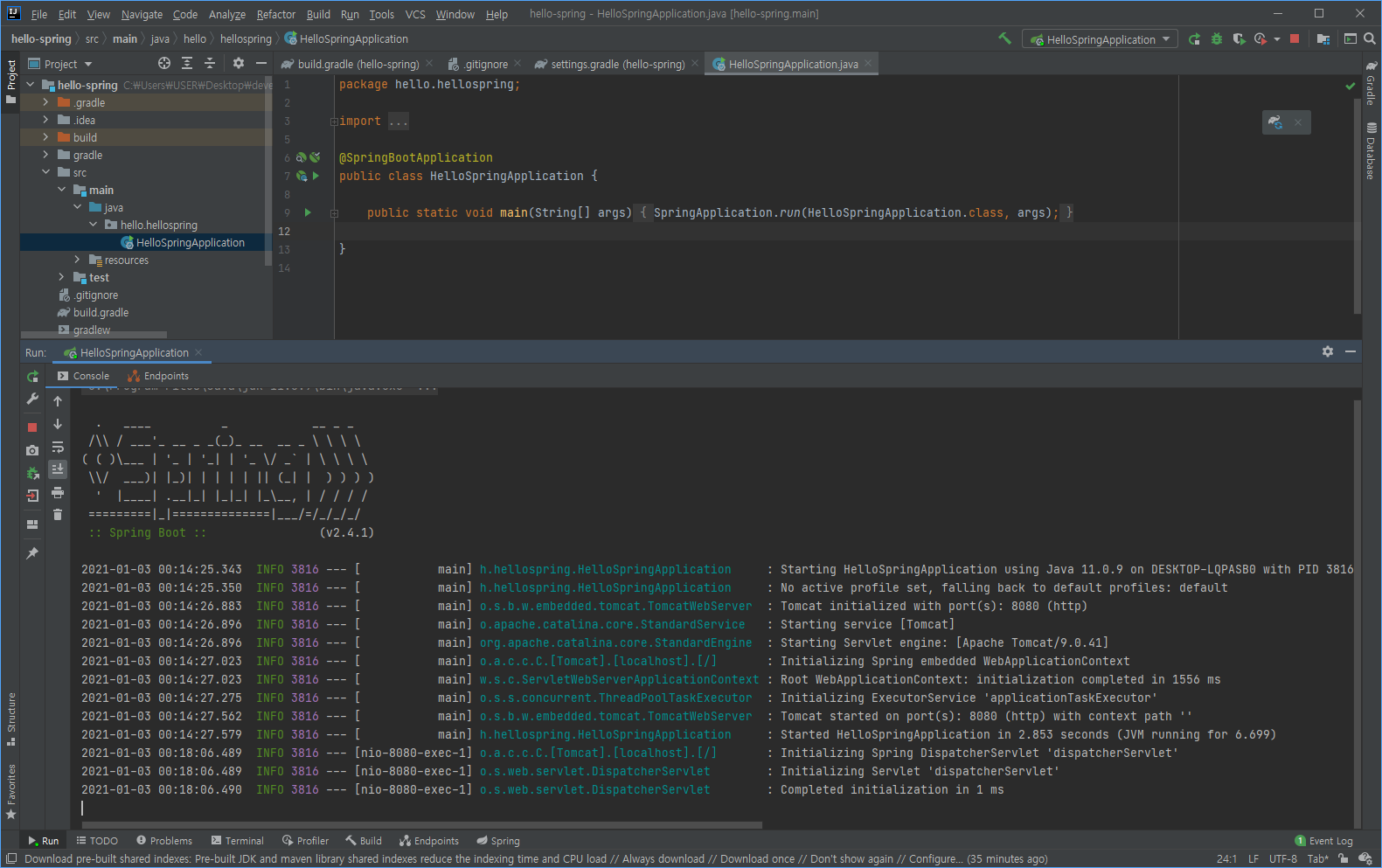

- 크롬으로 가서(다른 것도 ㄱㅊ), 주소창에 localhost:8080 을 입력하고 엔터를 눌러준다.
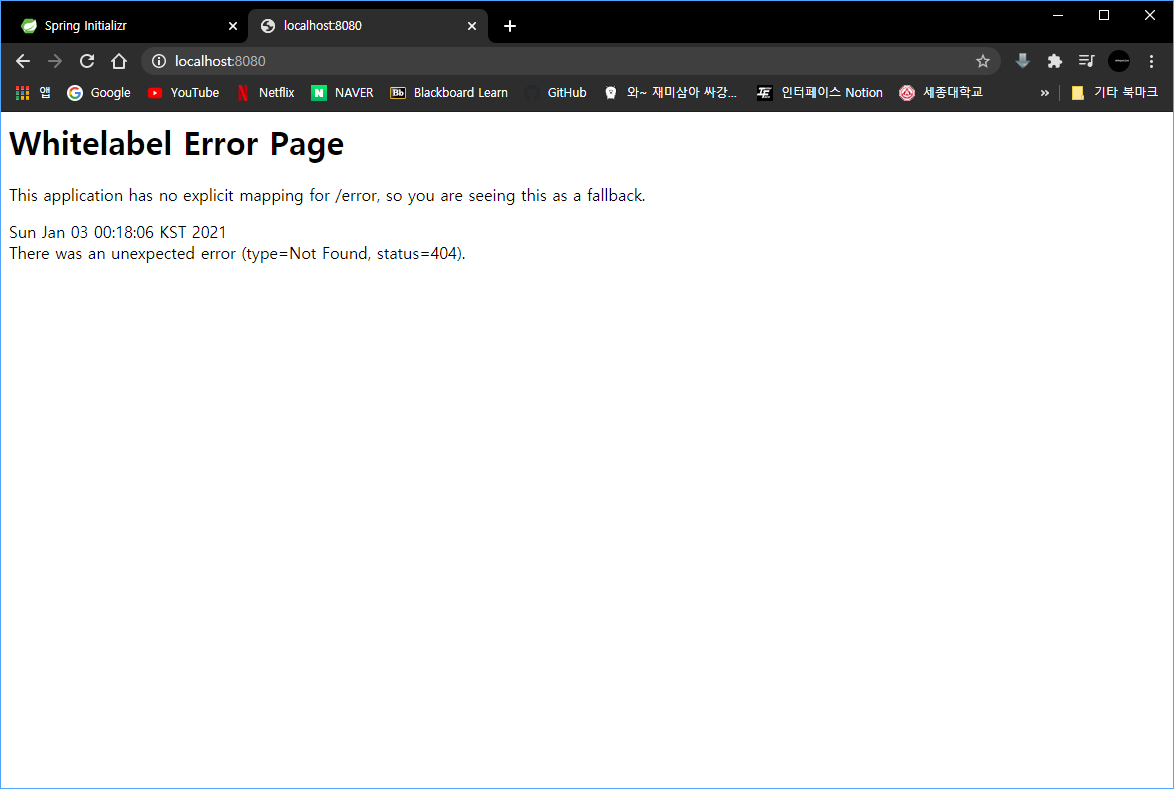
이렇게 뜰 것이다. (Run을 하지 않은 상태에서 접속하면 사이트에 연결할 수 없다는 문구가 뜬다.)
이제 Spring Boot에서 제공하는 Welcome Page 기능을 사용해보자.
공식문서를 살펴보면, 아래 설명처럼 index.html 파일을 만들어놓으면 자동으로 welcome page로 사용한다는 것을 알 수 있다.

- src > main > resources > static 에 index.html 파일을 추가한다.
1
2
3
4
5
6
7
8
9
10
11
|
<!DOCTYPE HTML>
<html>
<head>
<title>Hello</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8"/>
</head>
<body>
Hello
<a href="/hello">hello</a>
</body>
</html>
|
cs |
다시 localhost:8080으로 접속하면 아래와 같은 화면이 뜰 것이다.
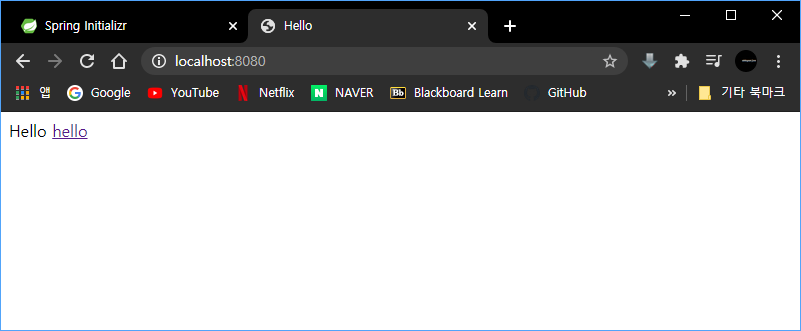
- src > main > java > hello.hellospring에 controller 라는 package를 생성한 후,
- HelloController 라는 이름의 클래스를 생성해준다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
package hello.hellospring.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloController {
@GetMapping("hello")
public String hello(Model model){
model.addAttribute("data", "hello!!");
return "hello";
}
}
|
cs |
- src > main > resources > templates에 hello.html 파일을 생성해준다.
1
2
3
4
5
6
7
8
9
10
|
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Hello</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
</head>
<body>
<p th:text="'안녕하세요. ' + ${data}" > 안녕하세요. 손님</p>
</body>
</html>
|
cs |
그리고 나서 http://localhost:8080/hello 를 실행하면
이렇게 된다 와~
1) hello 가 많아서 좀 헷갈려서, 파일을 조금씩 수정해서 다시 이해를 해보았다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
package hello.hellospring.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloController {
@GetMapping("hello")
public String something(Model model){
model.addAttribute("data", "spring!!");
return "hello";
}
}
|
cs |
@GetMapping("hello") << 이 hello는, hello.html 에서 Mapping 을 해주겠다는 뜻이다.
이 hello.html 에서 "data"가 있으면, 이걸 "spring!!"으로 바꿔준다.
이런 작업을 해준 다음에
return "hello" << 바뀐 hello.html을 return 해주는 것이다.
(hello를 다른 걸로 바꿔보았는데, 중 하나라도 바꾸면 에러 페이지가 뜬다.)
@GetMapping("hi")로 바꾸면 There was an unexpected error (type=Not Found, status=404).
return "hi" 로 바꾸면 There was an unexpected error (type=Internal Server Error, status=500).
2) hello.html 의 p 태그 안에 있는 '안녕하세요. 손님' 이 텍스트는 보이지 않는다.
그냥 딱 봤을 때 이런 형식으로 보이면 잘 작동 되는 것이라고 알기 위해 임시로 넣은 텍스트라고 생각하면 된다.
th.text를 이용해서, 그니까 thymeleaf 엔진을 사용해서 '안녕하세요. ' + ${data}, 이 내용으로 바뀌는 것이다.
지금은 그 data가 "spring!!"인 것이다.
1
2
3
4
5
6
7
8
9
10
|
<!DOCTYPE HTML>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Hello</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
</head>
<body>
<p th:text="'안녕하세요. ' + ${data}" > 어차피 안 보이지롱 </p>
</body>
</html>
|
cs |
이렇게 해놔도 localhost:8080/hello에서는 안 보인다. 안녕하세요 어쩌구로 대체된다.
3) http 는 8080 port를 쓴다.
예전이었으면 왜 8080이지 생각했을테데, 지금은 다행히도 컴퓨터 네트워크 수업시간에 배워서 알고있다.
컴네 수업 내용 다시 정리해보면서 다시 한 번 공부해봐야겠다...!
'etc > Spring' 카테고리의 다른 글
[Spring] MVC 와 템플릿 엔진 (0) | 2021.01.12 |
---|---|
[Spring] 정적 컨텐츠 (.html) 가져오기 (0) | 2021.01.04 |
[Spring] Port 8080 was already in use. (0) | 2021.01.04 |
[Spring] 프로젝트 빌드하고 실행하기 (0) | 2021.01.04 |
[Spring] spring-boot-devtools 라이브러리 추가하기 (0) | 2021.01.03 |
댓글
이 글 공유하기
다른 글
-
[Spring] 정적 컨텐츠 (.html) 가져오기
[Spring] 정적 컨텐츠 (.html) 가져오기
2021.01.04 -
[Spring] Port 8080 was already in use.
[Spring] Port 8080 was already in use.
2021.01.04 -
[Spring] 프로젝트 빌드하고 실행하기
[Spring] 프로젝트 빌드하고 실행하기
2021.01.04 -
[Spring] spring-boot-devtools 라이브러리 추가하기
[Spring] spring-boot-devtools 라이브러리 추가하기
2021.01.03